- Published on
Quantum Computing with Qiskit A Practical Introduction
- Authors
- Name
- Adil ABBADI
Introduction
Quantum computing is a revolutionary technology that has the potential to solve complex problems that are currently unsolvable with classical computers. In recent years, significant progress has been made in the development of quantum computers, and it's becoming increasingly important for developers to understand the basics of quantum computing and how to work with quantum systems.
In this blog post, we'll introduce you to the world of quantum computing and provide a practical guide to getting started with Qiskit, a popular open-source framework for quantum development.
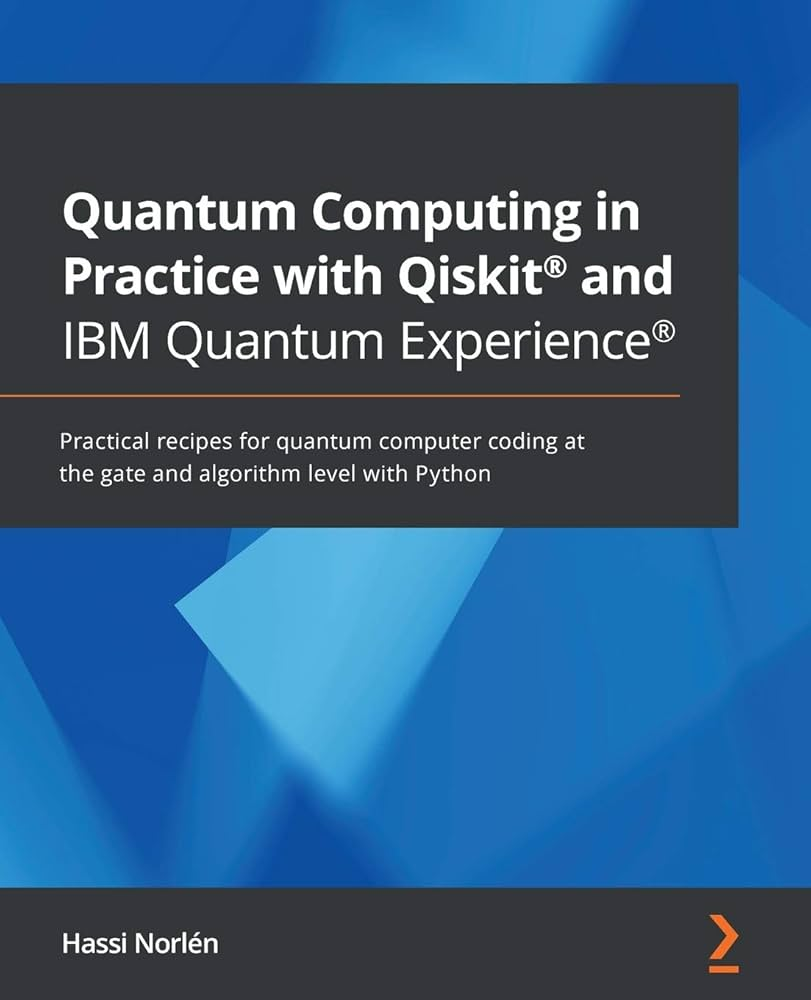
- What is Quantum Computing?
- What is Qiskit?
- Installing Qiskit
- Quantum Circuits with Qiskit
- Running Quantum Algorithms on Real Quantum Hardware
- Conclusion
- Ready to Start Your Quantum Journey?
What is Quantum Computing?
Quantum computing is a new paradigm for computing that uses the principles of quantum mechanics to perform operations on data. Unlike classical computers, which use bits to store and process information, quantum computers use quantum bits or qubits.
Qubits are unique because they can exist in multiple states simultaneously, which allows quantum computers to perform certain calculations much faster than classical computers. Quantum computing has many potential applications, including cryptography, optimization, and machine learning.
What is Qiskit?
Qiskit is an open-source framework for quantum development that provides a set of tools for researchers and developers to explore quantum computing. Qiskit was developed by IBM and is widely used in the quantum computing community.
Qiskit provides a range of features, including:
- A quantum development environment (QDE) for writing and executing quantum algorithms
- A set of pre-built quantum gates and circuits for constructing quantum algorithms
- Access to real quantum hardware through the IBM Quantum Experience
- A simulator for testing and debugging quantum algorithms
Installing Qiskit
To get started with Qiskit, you'll need to install it on your local machine. You can install Qiskit using pip:
pip install qiskit
Once you've installed Qiskit, you can verify the installation by running:
python -c "import qiskit; print(qiskit.__version__)"
This should display the version of Qiskit that you've installed.
Quantum Circuits with Qiskit
In Qiskit, quantum algorithms are represented as quantum circuits. A quantum circuit is a sequence of quantum gates that are applied to a set of qubits. Quantum gates are the quantum equivalent of logic gates in classical computing.
Here's an example of a simple quantum circuit in Qiskit:
from qiskit import QuantumCircuit, execute
# Create a quantum circuit with 2 qubits and 2 classical bits
circ = QuantumCircuit(2, 2)
# Add a H gate to qubit 0
circ.h(0)
# Add a CX gate to qubits 0 and 1
circ.cx(0, 1)
# Add a measurement to classical bits 0 and 1
circ.measure([0, 1], [0, 1])
# Execute the circuit
job = execute(circ, backend='qasm_simulator')
result = job.result()
print(result.get_counts(circ))
This circuit applies a Hadamard gate (H) to qubit 0, then applies a controlled-NOT gate (CX) to qubits 0 and 1. Finally, it measures the state of the qubits and stores the result in classical bits 0 and 1.
Running Quantum Algorithms on Real Quantum Hardware
One of the most exciting features of Qiskit is the ability to run quantum algorithms on real quantum hardware through the IBM Quantum Experience. To access the IBM Quantum Experience, you'll need to create an account and obtain an API token.
Here's an example of how to run the previous circuit on real quantum hardware:
from qiskit import QuantumCircuit, execute
from qiskit.providers.ibmq import least_busy
from qiskit.compiler import transpile
# Create a quantum circuit with 2 qubits and 2 classical bits
circ = QuantumCircuit(2, 2)
# Add a H gate to qubit 0
circ.h(0)
# Add a CX gate to qubits 0 and 1
circ.cx(0, 1)
# Add a measurement to classical bits 0 and 1
circ.measure([0, 1], [0, 1])
# Get the least busy backend
backend = least_busy(IBMQ.backends(simulator=False))
# Transpile the circuit for the backend
tcirc = transpile(circ, backend)
# Execute the circuit
job = execute(tcirc, backend=backend)
result = job.result()
print(result.get_counts(tcirc))
This code uses the least_busy
function to find the least busy backend, then transpiles the circuit for that backend using the transpile
function. Finally, it executes the circuit on the real quantum hardware and prints the result.
Conclusion
In this blog post, we've introduced you to the world of quantum computing and provided a practical guide to getting started with Qiskit. We've covered the basics of quantum computing, installed Qiskit, and written and executed a simple quantum circuit. We've also shown how to run quantum algorithms on real quantum hardware through the IBM Quantum Experience.
Quantum computing is a rapidly evolving field, and there's still much to be learned. However, with Qiskit, you have the tools you need to start exploring quantum computing today.
Ready to Start Your Quantum Journey?
Start learning quantum computing today and become proficient in using Qiskit for quantum development.