- Published on
C++ Memory Models Understanding Modern Hardware
- Authors
- Name
- Adil ABBADI
Introduction
As C++ continues to evolve, understanding the intricacies of memory models becomes increasingly important for developers. Modern hardware has introduced new complexities that require a deep comprehension of memory models to write efficient and correct code. In this blog post, we'll delve into the world of C++ memory models, exploring how they interact with modern hardware to optimize performance and ensure data consistency.
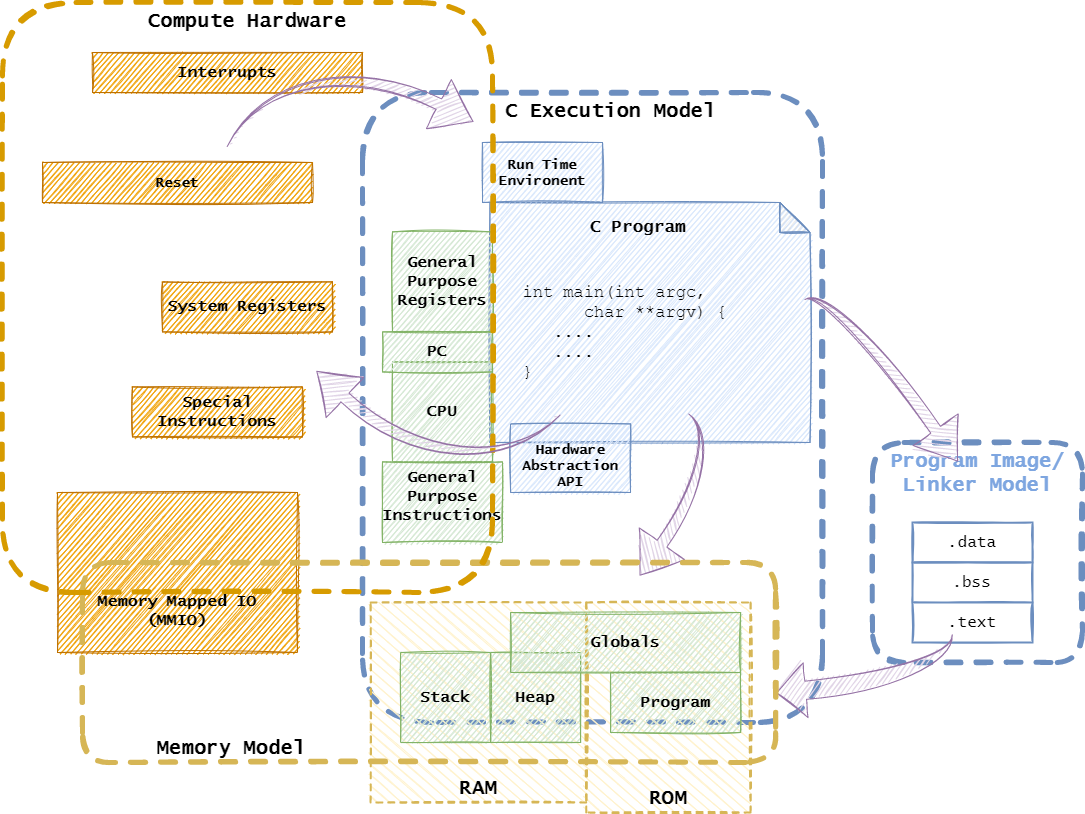
- The Basics of C++ Memory Models
- The C++ Memory Model and Modern Hardware
- Practical Example: Using C++ Memory Models with Modern Hardware
- Conclusion
- Ready to Dive Deeper into C++ Memory Models?
The Basics of C++ Memory Models
C++11 introduced a new memory model that defines how the language interacts with the underlying hardware. The C++ memory model consists of three primary components:
- Memory Locations: Each variable in a program has a unique memory location, which can be accessed using a pointer or reference.
- Accesses: An access is an operation that reads or writes to a memory location. There are two types of accesses: atomic and non-atomic.
- Atomic Operations: Atomic operations ensure that multiple threads can access the same memory location without interference.
The C++ Memory Model and Modern Hardware
Modern hardware, particularly multicore processors, has introduced new challenges for C++ memory models. To understand how C++ memory models interact with modern hardware, let's explore the following aspects:
Cache Coherence
In a multicore processor, each core has its own cache to improve performance. However, this leads to cache coherence issues, where multiple cores may have different values for the same memory location.
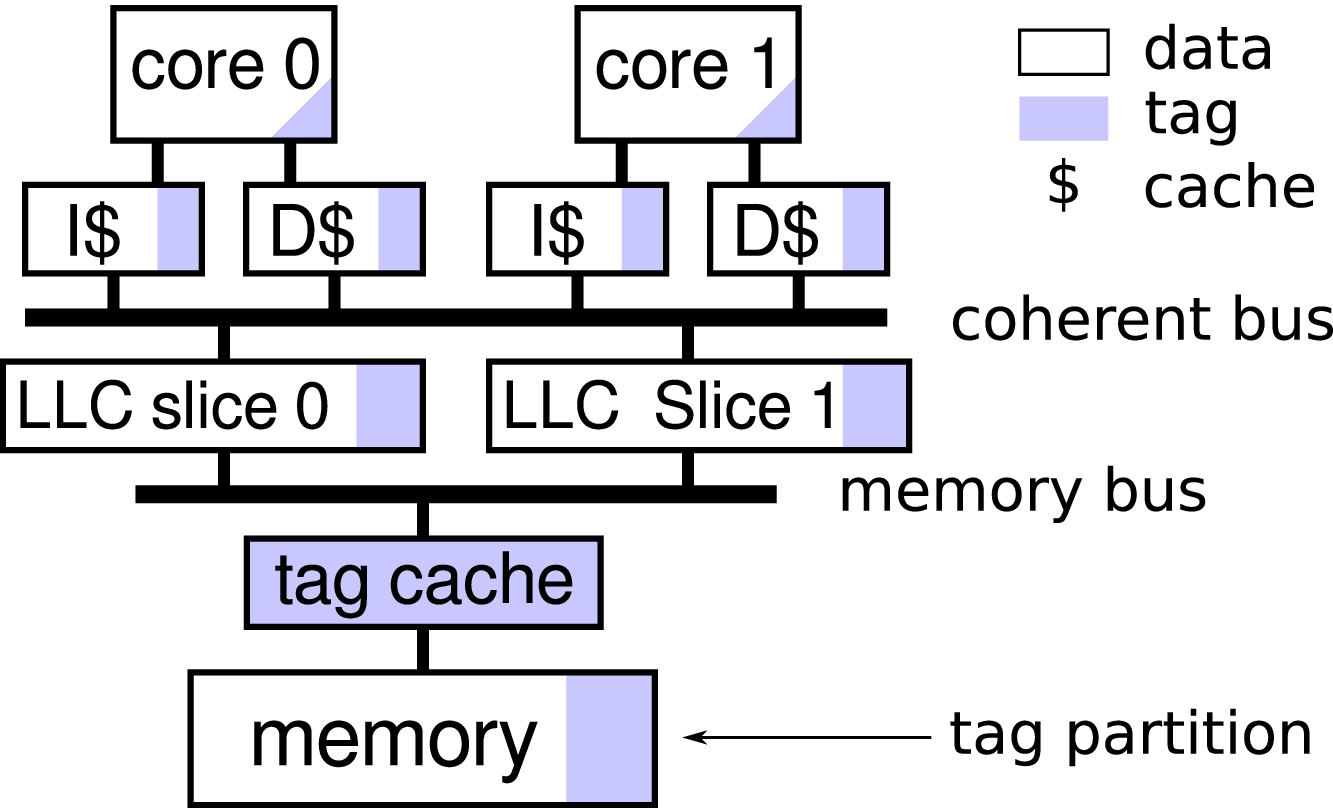
C++ memory models use atomic operations to ensure cache coherence. Atomic operations, such as std::atomic<int>
, use specialized instructions to update shared variables, ensuring that all cores see a consistent view of the data.
Memory Ordering
Memory ordering refers to the order in which memory accesses are executed. Modern hardware, particularly out-of-order execution processors, can execute instructions in a different order than they were written. C++ memory models provide several memory ordering options, such as:
- Sequential Consistency: Ensures that all accesses are executed in the order they were written.
- Acquire-Release Ordering: Ensures that all accesses are executed in the order they were written, with additional guarantees for atomic operations.
- Relaxed Ordering: Allows for more flexibility in memory ordering, but requires careful use to avoid data races.
Data Race Freedom
Data race freedom is a fundamental concept in C++ memory models. A data race occurs when multiple threads access the same memory location without proper synchronization, leading to unpredictable behavior.
C++ memory models provide several mechanisms to ensure data race freedom, including:
- Mutexes: Mutual exclusion locks that ensure only one thread can access a shared resource at a time.
- Atomic Operations: Use specialized instructions to update shared variables, ensuring that multiple threads can access the same memory location without interference.
- std::atomic: A C++ library that provides atomic operations and ensures data race freedom.
Practical Example: Using C++ Memory Models with Modern Hardware
Here is a practical example that demonstrates the use of C++ memory models with modern hardware:
#include <atomic>
#include <thread>
std::atomic<int> shared_variable(0);
void thread_func() {
for (int i = 0; i < 10000; ++i) {
shared_variable.fetch_add(1, std::memory_order_relaxed);
}
}
int main() {
std::thread t1(thread_func);
std::thread t2(thread_func);
t1.join();
t2.join();
std::cout << "Final value: " << shared_variable << std::endl;
return 0;
}
In this example, two threads increment a shared atomic variable 10,000 times each, demonstrating the use of C++ memory models to ensure data consistency and cache coherence.
Conclusion
C++ memory models are a crucial aspect of modern C++ development, and understanding how they interact with modern hardware is essential for writing efficient and correct code. By grasping the basics of C++ memory models and their interaction with modern hardware, developers can unlock the full potential of their code and ensure data consistency and performance.
Ready to Dive Deeper into C++ Memory Models?
Start exploring the intricacies of C++ memory models and modern hardware today, and take your C++ development skills to the next level.