- Published on
C++20 Coroutines Asynchronous Programming Made Simple
- Authors
- Name
- Adil ABBADI
Introduction
Asynchronous programming is an essential aspect of modern software development. It allows developers to write efficient, scalable, and responsive applications that can handle multiple tasks concurrently. In C++, asynchronous programming has traditionally been a complex and error-prone task, involving low-level threading APIs and callback-based programming models. However, with the introduction of C++20, a new era of asynchronous programming has begun. C++20 coroutines provide a simple, expressive, and efficient way to write asynchronous code, making it easier for developers to create concurrent programs.
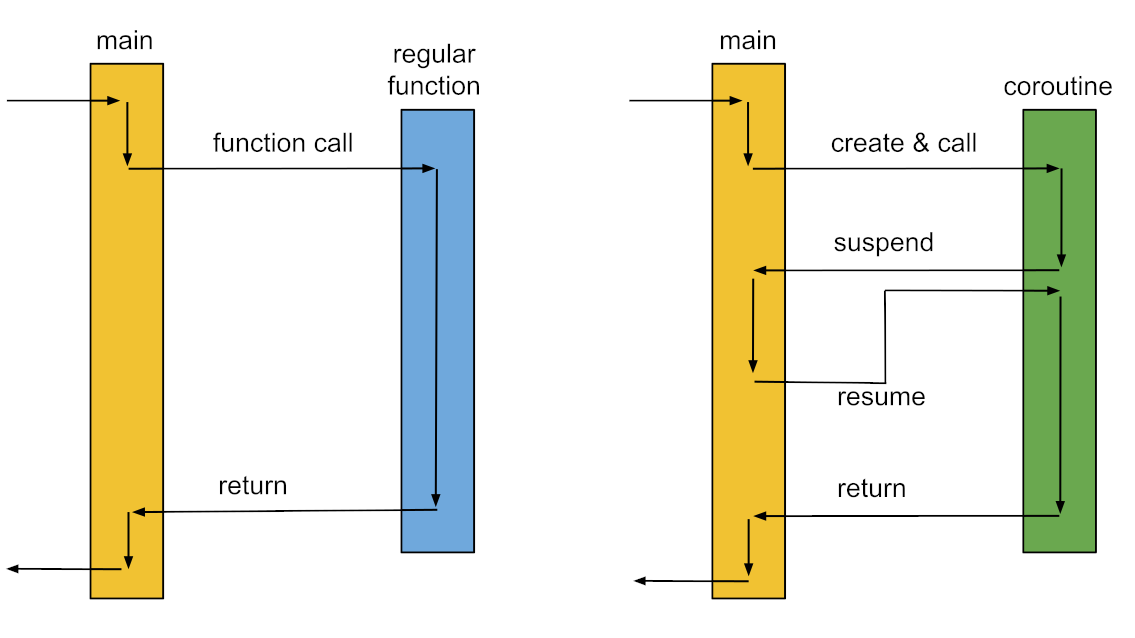
- What are Coroutines?
- C++20 Coroutines: A New Era of Asynchronous Programming
- Writing a Simple Coroutine
- Asynchronous Programming with Coroutines
- Benefits of C++20 Coroutines
- Conclusion
- Ready to Master C++20 Coroutines?
What are Coroutines?
Coroutines are a fundamental concept in computer science that allows a function to yield control to another function at specific points, allowing for efficient and lightweight context switching. In C++, coroutines are implemented as special functions that can suspend and resume their execution, enabling asynchronous programming.
C++20 Coroutines: A New Era of Asynchronous Programming
C++20 introduces a new set of language features that enable coroutine-based asynchronous programming. The key components of C++20 coroutines are:
co_await
andco_return
keywords: These keywords are used to define coroutine functions and to suspend and resume their execution.coroutine_handle
class: This class represents a coroutine object and provides methods for resuming, suspending, and destroying the coroutine.std::coroutine
namespace: This namespace provides a set of functions and classes for working with coroutines.
Writing a Simple Coroutine
Let's start with a simple example of a coroutine that prints a message to the console:
#include <coroutine>
#include <iostream>
std::coroutine_handle<> print_message() {
std::cout << "Hello, Coroutines!" << std::endl;
co_return;
}
int main() {
print_message();
return 0;
}
In this example, the print_message
function is a coroutine that prints a message to the console and then suspends its execution using the co_return
statement.
Asynchronous Programming with Coroutines
Now that we have a basic understanding of coroutines, let's dive into asynchronous programming. Suppose we want to write a program that fetches data from a network resource and processes it concurrently. Using C++20 coroutines, we can write a simple and efficient asynchronous program:
#include <coroutine>
#include <iostream>
#include <string>
#include <vector>
std::coroutine_handle<> fetch_data(const std::string& url) {
// simulate network request
std::this_thread::sleep_for(std::chrono::seconds(2));
std::string data = "data from " + url;
co_return data;
}
std::coroutine_handle<> process_data(const std::string& data) {
std::cout << "Processing data: " << data << std::endl;
co_return;
}
int main() {
auto data_handle = fetch_data("https://example.com/data");
auto process_handle = process_data(co_await data_handle);
// wait for both coroutines to complete
data_handle.wait();
process_handle.wait();
return 0;
}
In this example, we define two coroutines: fetch_data
and process_data
. The fetch_data
coroutine simulates a network request and returns the fetched data. The process_data
coroutine takes the data as an input and processes it concurrently. We use the co_await
keyword to suspend the execution of the main
function until the fetch_data
coroutine completes and returns the data.
Benefits of C++20 Coroutines
C++20 coroutines provide several benefits that make asynchronous programming easier and more efficient:
- Simplified Code: Coroutines simplify the code and make it more readable by eliminating the need for low-level threading APIs and callback-based programming models.
- Efficient Context Switching: Coroutines enable efficient context switching, which reduces the overhead of concurrent programming.
- Improved Responsiveness: Coroutines allow for responsive and interactive applications that can handle multiple tasks concurrently.
Conclusion
C++20 coroutines are a game-changer for concurrent programming in C++. They provide a simple, expressive, and efficient way to write asynchronous code, making it easier for developers to create concurrent programs. With coroutines, developers can write more efficient, scalable, and responsive applications that can handle multiple tasks concurrently.
Ready to Master C++20 Coroutines?
Start exploring the world of C++20 coroutines today and discover the power of asynchronous programming in C++.
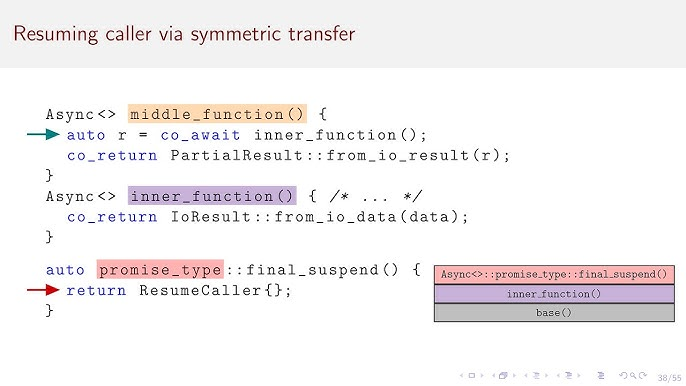