- Published on
Building Custom Chatbots with OpenAI API A Complete Guide
- Authors
- Name
- Adil ABBADI
Introduction
Chatbots have revolutionized the way businesses interact with their customers, providing 24/7 support and automating routine tasks. However, building a custom chatbot from scratch can be a daunting task, requiring extensive knowledge of natural language processing (NLP) and machine learning. Fortunately, the OpenAI API provides a powerful tool for building custom chatbots with ease. In this comprehensive guide, we'll explore how to build a custom chatbot using the OpenAI API, covering the basics, implementation, and best practices.

What is the OpenAI API?
The OpenAI API is a powerful tool for natural language processing, providing a simple and intuitive interface for building AI-powered applications. It offers a range of models and algorithms for tasks such as language translation, text classification, and conversation generation. The OpenAI API is built on top of the OpenAI GPT-3 model, a state-of-the-art language model that has been trained on a massive dataset of text from the internet.
Why Use the OpenAI API for Building Chatbots?
There are several reasons why the OpenAI API is an ideal choice for building custom chatbots:
- Ease of use: The OpenAI API provides a simple and intuitive interface, requiring minimal knowledge of NLP and machine learning.
- Powerful models: The OpenAI API is built on top of the OpenAI GPT-3 model, which is one of the most advanced language models available.
- Customizability: The OpenAI API allows you to customize your chatbot to suit your specific needs and goals.
Setting Up the OpenAI API
Before we dive into building our chatbot, let's set up the OpenAI API:
- Create an account: Sign up for an account on the OpenAI website.
- Get an API key: Once you've signed up, you'll receive an API key.
- Install the OpenAI library: Install the OpenAI library using pip:
pip install openai
Building a Simple Chatbot
Now that we've set up the OpenAI API, let's build a simple chatbot:
import openai
# Set up the OpenAI API client
openai.api_key = "YOUR_API_KEY"
# Define the chatbot's personality and tone
personality = "friendly"
tone = "helpful"
# Define the chatbot's response function
def respond_to_user(input_text):
response = openai.Completion.create(
engine="davinci",
prompt=input_text,
max_tokens=100,
temperature=0.5,
top_p=1,
frequency_penalty=0,
presence_penalty=0
)
return response.choices[0].text
# Test the chatbot
user_input = "Hello, how are you?"
response = respond_to_user(user_input)
print(response)
This code sets up the OpenAI API client, defines the chatbot's personality and tone, and defines a response function that uses the OpenAI API to generate a response to the user's input.
Implementing Advanced Features
Now that we've built a simple chatbot, let's implement some advanced features:
Contextual Understanding
To improve our chatbot's ability to understand context, we can use the OpenAI API's Conversational
engine:
def respond_to_user(input_text, conversation_history):
response = openai.Conversation.create(
engine="conversational",
prompt=input_text,
conversation_history=conversation_history,
max_tokens=100,
temperature=0.5,
top_p=1,
frequency_penalty=0,
presence_penalty=0
)
return response.choices[0].text
This code uses the Conversational
engine to generate a response based on the user's input and conversation history.
Intent Identification
To identify the user's intent, we can use the OpenAI API's TextClassification
engine:
def identify_intent(input_text):
response = openai.TextClassification.create(
engine="text-classification",
prompt=input_text,
labels=["greeting", "goodbye", "help"]
)
return response.label
This code uses the TextClassification
engine to identify the user's intent based on their input.
Integration with External APIs
To integrate our chatbot with external APIs, we can use the requests
library in Python:
import requests
def get_weather_data(city):
response = requests.get(f"http://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q={city}")
return response.json()
This code uses the requests
library to retrieve weather data from an external API.
Deployment and Testing
Once we've built our chatbot, we need to deploy and test it. There are several ways to deploy a chatbot, including:
- Web interface: Create a web interface using Flask or Django.
- Messaging platform: Integrate with messaging platforms like Facebook Messenger or WhatsApp.
- Voice assistants: Integrate with voice assistants like Alexa or Google Assistant.
Best Practices for Building Chatbots
Here are some best practices for building chatbots:
- Define clear goals and objectives: Determine what you want your chatbot to achieve.
- Understand your target audience: Understand who your chatbot will be interacting with.
- Keep it simple and intuitive: Ensure that your chatbot is easy to use and understand.
- Test and iterate: Continuously test and iterate your chatbot to improve its performance.
Conclusion
In this comprehensive guide, we've explored how to build a custom chatbot using the OpenAI API. We've covered the basics of the OpenAI API, implementation, and best practices. With the OpenAI API, you can build powerful and customize chatbots that revolutionize user interactions.
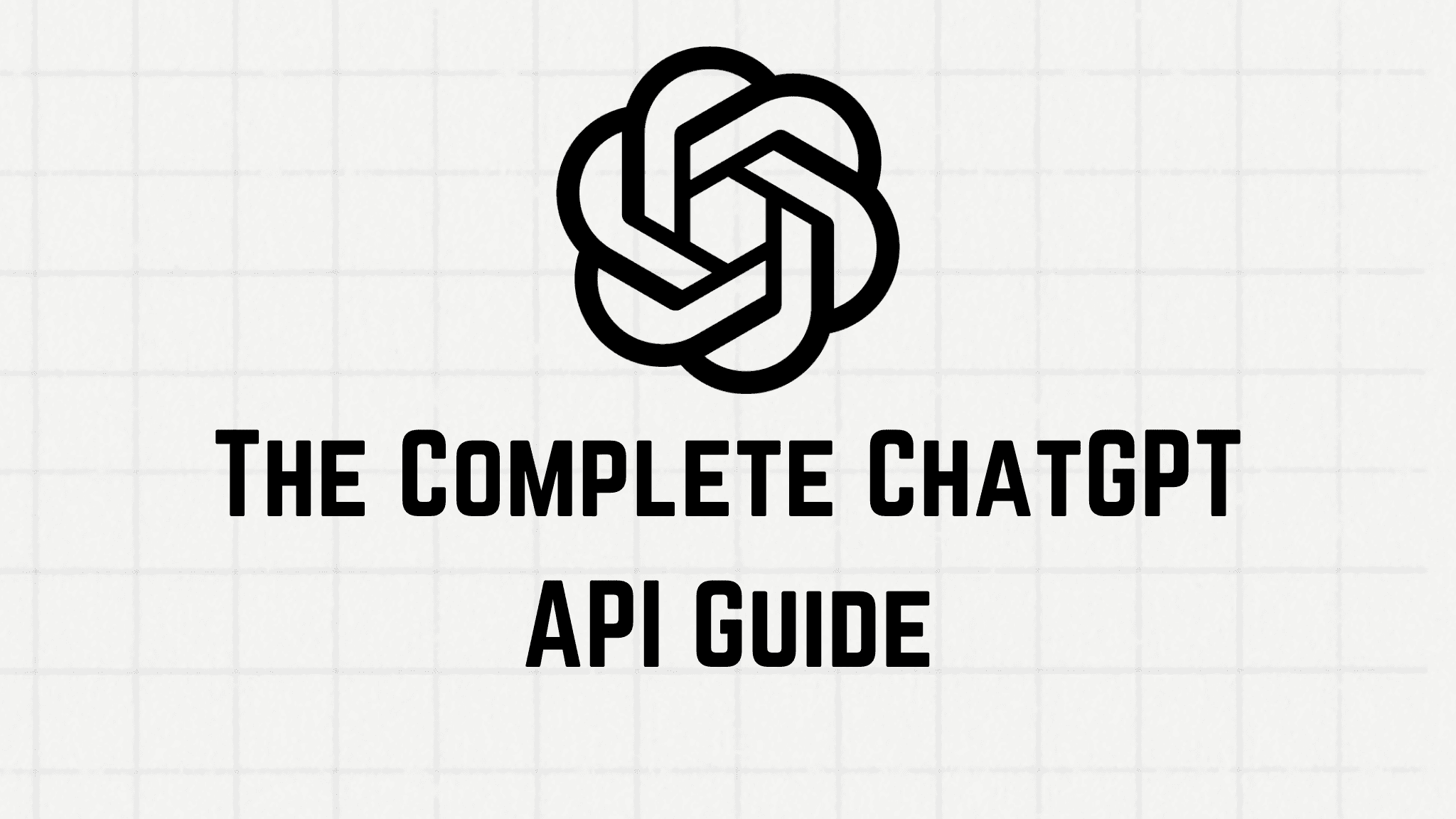
Ready to start building your custom chatbot? Sign up for an OpenAI API key today and start creating!
Do not include any introductory text.