- Published on
C++ Template Metaprogramming Advanced Techniques
- Authors
- Name
- Adil ABBADI
Introduction
C++ template metaprogramming is a powerful feature that allows developers to generate code at compile-time. It enables the creation of generic and flexible code that can be used in a wide range of applications. In this blog post, we'll dive into advanced template metaprogramming techniques that will take your C++ skills to the next level.
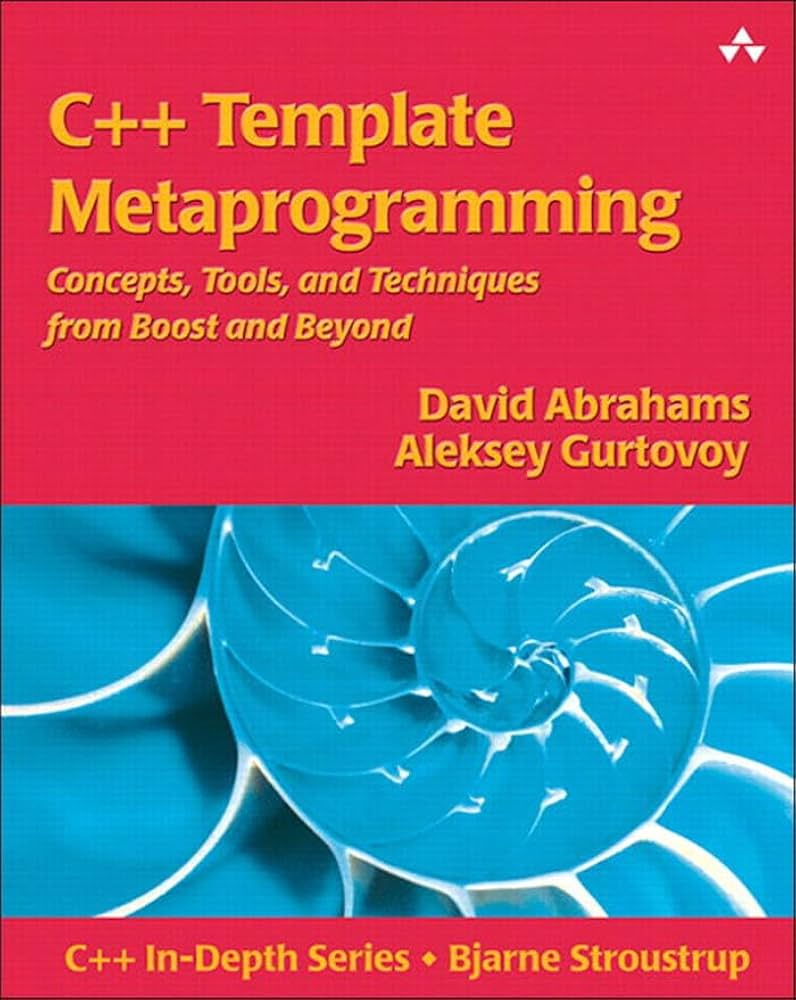
- Advanced Template Metaprogramming Concepts
- Advanced Techniques
- Conclusion
- Ready to Take Your C++ Skills to the Next Level?
Advanced Template Metaprogramming Concepts
Before we dive into the advanced techniques, let's review some essential concepts:
- Template recursion: A technique used to generate code at compile-time by recursively instantiating templates.
- Template parameter packs: A way to pass a variable number of template arguments to a template.
- SFINAE (Substitution Failure Is Not An Error): A technique used to selectively disable or enable function overloads based on the validity of the template instantiation.
1. Metafunctions
A metafunction is a function that operates on types at compile-time. It's a fundamental concept in template metaprogramming. Here's an example of a metafunction that calculates the sum of a variadic number of integers:
template <typename... Ts>
struct sum {
static constexpr int value = (Ts::value + ...);
};
template <>
struct sum<> {
static constexpr int value = 0;
};
2. Type Traits
Type traits are metafunctions that provide information about a type. They're essential in template metaprogramming. Here's an example of a type trait that checks if a type is a pointer:
template <typename T>
struct is_pointer {
static constexpr bool value = false;
};
template <typename T>
struct is_pointer<T*> {
static constexpr bool value = true;
};
3. SFINAE with std::enable_if
SFINAE is a powerful technique used to selectively disable or enable function overloads based on the validity of the template instantiation. Here's an example of using SFINAE with std::enable_if
to enable a function only if the type is a pointer:
template <typename T>
std::enable_if_t<std::is_pointer_v<T>, void> foo(T t) {
// code that only works with pointers
}
4. Variadic Templates
Variadic templates allow you to pass a variable number of template arguments to a template. Here's an example of a variadic template that forwards its arguments to another function:
template <typename... Ts>
void forward(Ts&&... args) {
some_function(std::forward<Ts>(args)...);
}
5. Template Metaprogramming with Lambdas
C++14 introduced lambda expressions, which can be used in conjunction with template metaprogramming. Here's an example of using a lambda to create a metafunction:
auto add = [](auto x, auto y) {
return x + y;
};
template <typename T>
struct add_t {
static constexpr T value = add(T{}, T{});
};
Advanced Techniques
Now that we've covered the basics, let's dive into some advanced template metaprogramming techniques:
1. Compile-Time Evaluation of Mathematical Expressions
Template metaprogramming allows you to evaluate mathematical expressions at compile-time. Here's an example of calculating the factorial of a number at compile-time:
template <unsigned int N>
struct factorial {
static constexpr unsigned int value = N * factorial<N - 1>::value;
};
template <>
struct factorial<0> {
static constexpr unsigned int value = 1;
};
2. Code Generation with Template Metaprogramming
Template metaprogramming can be used to generate code at compile-time. Here's an example of generating a sequence of numbers at compile-time:
template <unsigned int N>
struct sequence {
static constexpr unsigned int value = N;
using next = sequence<N - 1>;
};
template <>
struct sequence<0> {
static constexpr unsigned int value = 0;
};
3. Domain-Specific Languages (DSLs) with Template Metaprogramming
Template metaprogramming can be used to create domain-specific languages (DSLs) that allow you to express complex logic in a concise and expressive way. Here's an example of creating a DSL for matrix operations:
template <typename Mat1, typename Mat2>
struct mat_add {
using type = matrix/mat_add_impl<Mat1, Mat2>>>;
};
template <typename Mat1, typename Mat2>
struct mat_add_impl {
// implementation of matrix addition
};
4. Compile-Time String Processing
Template metaprogramming can be used to process strings at compile-time. Here's an example of converting a string to uppercase at compile-time:
template <char... Cs>
struct to_uppercase {
static constexpr char value[] = {std::toupper(Cs)...};
};
5. Advanced SFINAE Techniques
SFINAE is a powerful technique that allows you to selectively disable or enable function overloads based on the validity of the template instantiation. Here's an example of using SFINAE to create a function that only works with arithmetic types:
template <typename T, typename = std::enable_if_t<std::is_arithmetic_v<T>>>
void foo(T t) {
// code that only works with arithmetic types
}
Conclusion
In this blog post, we've explored advanced template metaprogramming techniques in C++. By mastering these techniques, you'll be able to create more efficient, flexible, and expressive code that takes advantage of C++'s compile-time evaluation capabilities.
Ready to Take Your C++ Skills to the Next Level?
Start exploring the world of template metaprogramming today and discover the power of generating code at compile-time.