- Published on
Unlocking Efficiency Context Window Optimization for Large Language Models
- Authors
- Name
- Adil ABBADI
Introduction
As large language models continue to shape the landscape of natural language processing (NLP), it's essential to address the critical aspect of context window optimization. The ever-growing size of these models demands efficient processing, and optimizing the context window is crucial to achieving this goal. In this article, we'll explore the significance of context window optimization, its challenges, and provide actionable strategies for improving the performance of large language models.
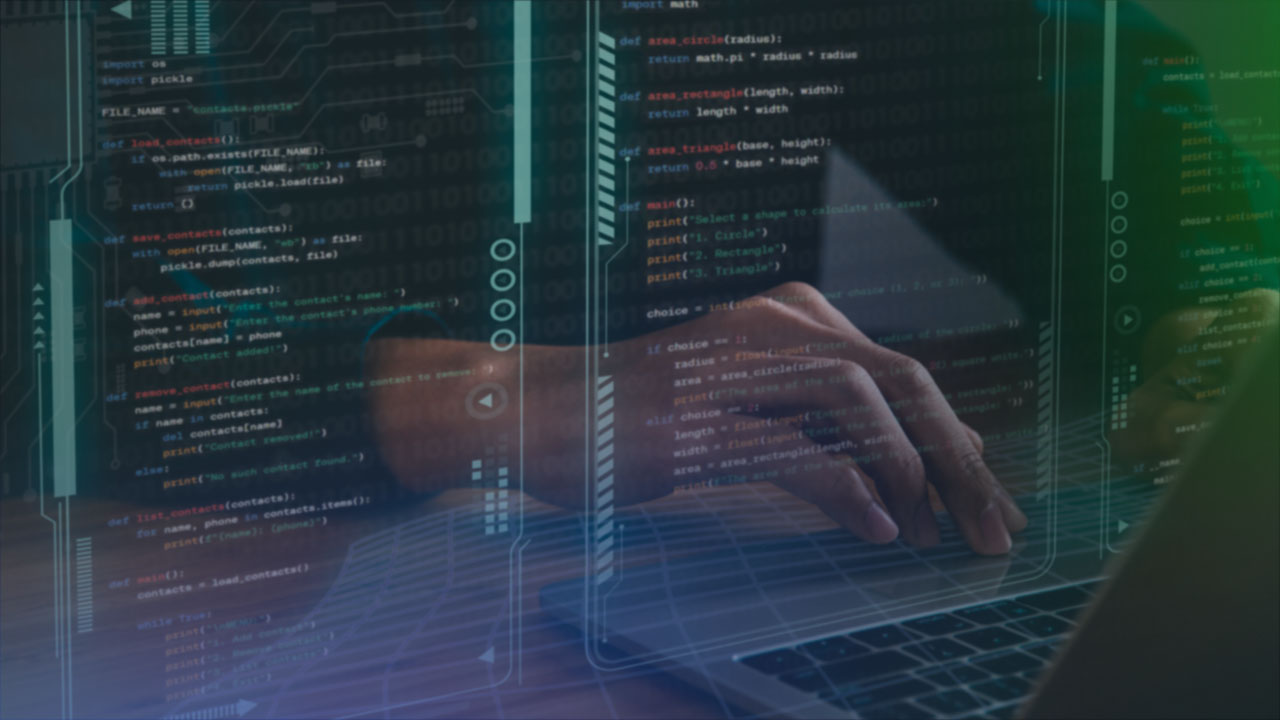
- Understanding Context Windows
- Challenges in Context Window Optimization
- Strategies for Context Window Optimization
- Conclusion
- Further Exploration
Understanding Context Windows
In large language models, the context window refers to the span of input tokens considered when processing a given token. The size of the context window directly impacts the model's performance, memory usage, and computational resources required. A larger context window can capture more nuanced contextual relationships, but it also increases computational costs.
import torch
from transformers import AutoModelForSequenceClassification, AutoTokenizer
# Load the pre-trained model and tokenizer
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased")
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
# Define a sample input sequence
input_sequence = "This is an example sentence."
# Tokenize the input sequence
inputs = tokenizer.encode_plus(
input_sequence,
add_special_tokens=True,
max_length=512,
return_attention_mask=True,
return_tensors="pt"
)
# Set the context window size
context_window_size = 128
# Process the input sequence with the specified context window size
output = model(inputs["input_ids"], attention_mask=inputs["attention_mask"], context_window_size=context_window_size)
Challenges in Context Window Optimization
Optimizing the context window is a delicate balance between capturing relevant context and managing computational resources. Some of the challenges include:
- Computational Cost: Larger context windows lead to increased computational costs, making it essential to find the sweet spot between context and performance.
- Memory Constraints: Models with large context windows require significant memory, which can be a limitation for deployment on resource-constrained devices.
- Contextual Noise: Increased context window sizes can introduce noise, reducing the model's overall performance.
Strategies for Context Window Optimization
1. Dynamic Context Window
Adopt a dynamic context window approach, where the window size is adaptively adjusted based on the input sequence. This approach can help balance computational costs and contextual information.
def dynamic_context_window(input_sequence, context_window_size):
# Calculate the optimal context window size based on input sequence length
optimal_size = min(context_window_size, len(input_sequence) // 2)
return optimal_size
2. Hierarchical Context Window
Implement a hierarchical context window approach, where the model processes the input sequence in a hierarchical manner, with smaller context windows for earlier layers and larger windows for later layers.
class HierarchicalContextWindow(nn.Module):
def __init__(self, context_window_sizes):
super(HierarchicalContextWindow, self).__init__()
self.context_window_sizes = context_window_sizes
def forward(self, input_sequence):
# Process the input sequence with hierarchical context windows
for i, size in enumerate(self.context_window_sizes):
output = self.layers[i](input_sequence, context_window_size=size)
input_sequence = output
return output
3. Context Window Pruning
Employ context window pruning techniques to eliminate redundant or irrelevant context tokens, reducing computational costs and improving performance.
def context_window_pruning(input_sequence, context_window_size, threshold):
# Calculate attention scores for each token in the context window
attention_scores = model(input_sequence, context_window_size=context_window_size).attention_scores
# Prune tokens with attention scores below the threshold
pruned_sequence = [token for token, score in zip(input_sequence, attention_scores) if score > threshold]
return pruned_sequence
Conclusion
Context window optimization is a critical aspect of large language model performance. By understanding the challenges and implementing strategies such as dynamic context windows, hierarchical context windows, and context window pruning, developers can unlock efficiency and improve the performance of these models.
Further Exploration
Explore the realm of context window optimization further by experimenting with different techniques and evaluating their impact on your own large language models. Remember to consider the trade-offs between computational costs, memory usage, and contextual information when optimizing the context window.
Happy optimizing!