- Published on
Smart Pointers in Modern C++ Memory Management Best Practices
- Authors
- Name
- Adil ABBADI
Introduction
Memory management is a crucial aspect of C++ programming, and with the advent of modern C++, smart pointers have become an essential tool for managing memory efficiently and safely. In this blog post, we will delve into the world of smart pointers, exploring their benefits, best practices, and common pitfalls to avoid.
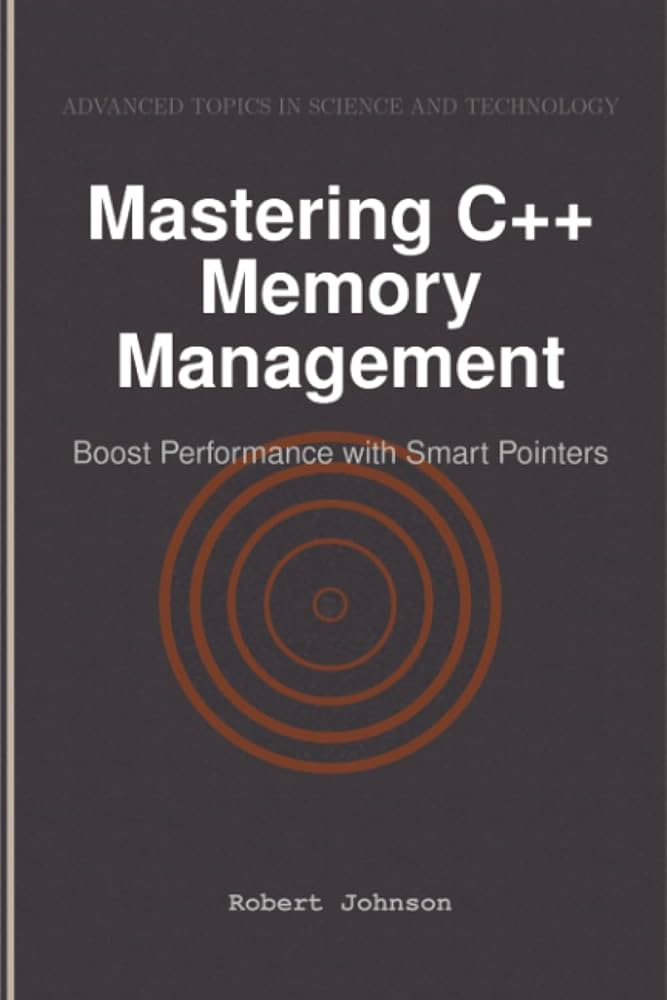
- What are Smart Pointers?
- Unique Pointers (std::unique_ptr)
- Shared Pointers (std::shared_ptr)
- Weak Pointers (std::weak_ptr)
- Best Practices for Using Smart Pointers
- Common Pitfalls to Avoid
- Conclusion
What are Smart Pointers?
Smart pointers are a type of C++ class that provide automatic memory management for dynamically allocated objects. They are designed to replace traditional raw pointers, which can lead to memory leaks, dangling pointers, and other issues. There are three types of smart pointers in modern C++: unique_ptr
, shared_ptr
, and weak_ptr
.
Unique Pointers (std::unique_ptr)
std::unique_ptr
is a smart pointer that owns and manages a single object or array. It ensures that the object is deleted when the unique_ptr
goes out of scope or is reset. Unique pointers are useful when you need to manage a single object and ensure that it is not copied or shared.
#include <memory>
class Foo {
public:
Foo() { std::cout << "Foo constructed\n"; }
~Foo() { std::cout << "Foo destroyed\n"; }
};
int main() {
std::unique_ptr<Foo> foo = std::make_unique<Foo>();
return 0;
}
Shared Pointers (std::shared_ptr)
std::shared_ptr
is a smart pointer that allows multiple pointers to share ownership of a single object. The object is deleted when the last shared pointer to it is destroyed. Shared pointers are useful when you need to share objects between multiple parts of your code.
#include <memory>
class Foo {
public:
Foo() { std::cout << "Foo constructed\n"; }
~Foo() { std::cout << "Foo destroyed\n"; }
};
int main() {
std::shared_ptr<Foo> foo1 = std::make_shared<Foo>();
std::shared_ptr<Foo> foo2 = foo1;
return 0;
}
Weak Pointers (std::weak_ptr)
std::weak_ptr
is a smart pointer that allows observing an object managed by a shared pointer without taking ownership. Weak pointers are useful when you need to break circular dependencies between objects.
#include <memory>
class Foo {
public:
Foo() { std::cout << "Foo constructed\n"; }
~Foo() { std::cout << "Foo destroyed\n"; }
};
int main() {
std::shared_ptr<Foo> foo = std::make_shared<Foo>();
std::weak_ptr<Foo> weakFoo = foo;
return 0;
}
Best Practices for Using Smart Pointers
- Use smart pointers instead of raw pointers: Smart pointers provide automatic memory management, which reduces the risk of memory leaks and dangling pointers.
- Use
std::make_unique
andstd::make_shared
: These functions provide a safe and efficient way to create smart pointers. - Avoid naked
new
anddelete
: Nakednew
anddelete
can lead to memory leaks and should be avoided in favor of smart pointers. - Use
std::weak_ptr
to break circular dependencies: Weak pointers help prevent circular dependencies between objects, which can lead to memory leaks. - Avoid using raw pointers as function parameters: Instead, use smart pointers or references to ensure safe and efficient memory management.
Common Pitfalls to Avoid
- Dangling Pointers: A dangling pointer points to memory that has already been deleted. Smart pointers help avoid dangling pointers by automatically managing memory.
- Circular Dependencies: Circular dependencies occur when two or more objects reference each other, leading to memory leaks. Weak pointers can help break circular dependencies.
- Memory Leaks: Memory leaks occur when memory is allocated but not released. Smart pointers help prevent memory leaks by automatically managing memory.
- ** Double-Free**: A double-free occurs when the same memory is deleted twice. Smart pointers help prevent double-free by ensuring that memory is deleted only once.
Conclusion
Smart pointers are an essential tool in modern C++ for efficient and safe memory management. By following best practices and avoiding common pitfalls, you can write robust and efficient C++ code that minimizes memory-related issues.
Ready to master smart pointers in modern C++? Start improving your C++ skills today and become proficient in using smart pointers for efficient memory management.