- Published on
Rust's Async/Await Modern Concurrency Patterns
- Authors
- Name
- Adil ABBADI
Introduction
In today's world of software development, concurrency is an essential aspect of building efficient and scalable systems. Rust, being a systems programming language, provides a robust concurrency model that allows developers to write fast, reliable, and concurrent code. In this blog post, we'll delve into Rust's async/await system and explore modern concurrency patterns.
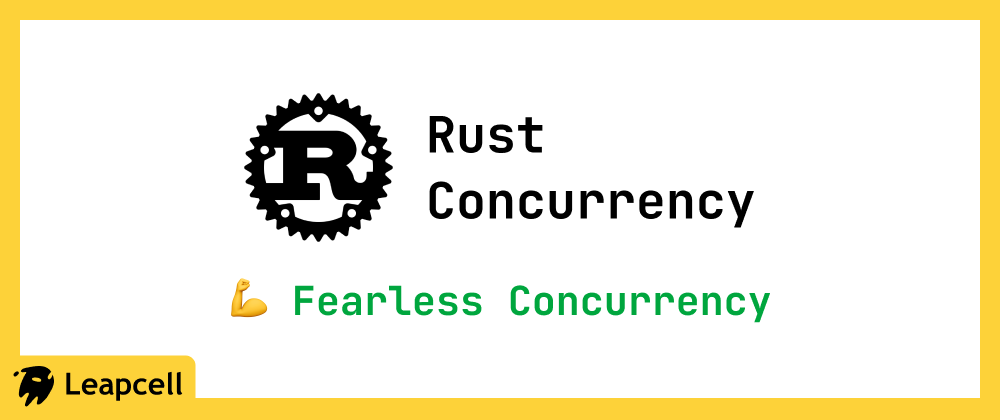
- Understanding Rust's Concurrency Model
- Async/Await: A Modern Concurrency Pattern
- Tokio: A Rust Async Runtime
- Best Practices for Async/Await
- Conclusion
- Ready to Master Async/Await?
Understanding Rust's Concurrency Model
Rust's concurrency model is built around the concept of ownership and borrowing. The language's ownership system ensures memory safety, while its borrowing system allows for flexible and efficient concurrency. Rust provides two primary concurrency primitives: std::thread
and std::async
.
std::thread
std::thread
provides a low-level, blocking API for creating threads. While it's a viable option for concurrency, it can be error-prone and lead to performance issues.
std::async
std::async
, on the other hand, provides a high-level, non-blocking API for writing asynchronous code. It's built on top of the Future
trait, which represents a computation that may not have completed yet.
Async/Await: A Modern Concurrency Pattern
Async/await is a modern concurrency pattern that simplifies writing asynchronous code. It's built on top of std::async
and provides a more readable and maintainable way of writing concurrent code.
Async Functions
Async functions are special functions that return a Future
instance. They're marked with the async
keyword and can contain await
expressions.
async fn my_async_function() {
// async code here
}
Await Expressions
Await expressions are used to pause the execution of an async function until the Future
instance is resolved. They're marked with the await
keyword.
async fn my_async_function() {
let future = async { /* some async operation */ };
let result = await!(future);
// process result
}
Async/Await in Practice
Let's create a simple async/await example that demonstrates a concurrent HTTP request:
use reqwest::async::Client;
async fn fetch_data(url: &str) -> Result<String, reqwest::Error> {
let client = Client::new();
let res = client.get(url).await?;
let body = res.text().await?;
Ok(body)
}
#[tokio::main]
async fn main() {
let url = "https://example.com";
let data = fetch_data(url).await?;
println!("{}", data);
}
In this example, we define an fetch_data
async function that performs a GET request using the reqwest
library. We then call fetch_data
from the main
function, using the await
expression to wait for the result.
Tokio: A Rust Async Runtime
Tokio is a popular async runtime for Rust that provides a robust and efficient way of running asynchronous code. It's built on top of the std::async
API and provides a rich set of features for concurrent programming.
Tokio's Core Abstractions
Tokio provides three core abstractions:
- Task: A unit of asynchronous execution.
- Sender: A channel for sending values between tasks.
- Receiver: A channel for receiving values between tasks.
Tokio in Practice
Let's create a Tokio example that demonstrates a concurrent ping-pong game:
use tokio::{prelude::*, task};
async fn ping_pong() {
let (tx, mut rx) = tokio::sync::mpsc::channel(10);
task::spawn(async move {
(tx.send("ping").await.unwrap();
});
while let Some(msg) = rx.recv().await {
println!("{}", msg);
if msg == "ping" {
tx.send("pong").await.unwrap();
} else {
tx.send("ping").await.unwrap();
}
}
}
#[tokio::main]
async fn main() {
ping_pong().await;
}
In this example, we define a ping_pong
async function that creates a Tokio channel for sending and receiving messages. We then spawn a task that sends a "ping" message, and use a while
loop to receive and respond to messages.
Best Practices for Async/Await
Here are some best practices to keep in mind when using async/await in Rust:
- Use
async fn
instead offn
: Clearly indicate that a function is asynchronous. - Use
await?
instead ofawait
: Propagate errors using the?
operator. - Avoid blocking code: Use non-blocking APIs whenever possible.
- Use Tokio for complex concurrency: Leverage Tokio's abstractions for robust and efficient concurrency.
Conclusion
In this blog post, we explored Rust's async/await system and modern concurrency patterns. We discussed the basics of async/await, Tokio's core abstractions, and best practices for writing concurrent code. By mastering async/await and Tokio, you can build fast, reliable, and scalable systems in Rust.
Ready to Master Async/Await?
Start improving your concurrency skills today and become proficient in using async/await and Tokio for robust concurrent programming.