- Published on
Rust for Embedded Systems Safe Systems Programming
- Authors
- Name
- Adil ABBADI
Introduction
In the realm of embedded systems, reliability and safety are paramount. A single fault or error can have catastrophic consequences, resulting in system failures, financial losses, and even loss of life. Traditionally, C and C++ have been the languages of choice for embedded systems development. However, these languages' lack of memory safety guarantees and error-prone nature make them less than ideal for modern embedded systems.
Rust, a systems programming language, offers a unique solution to these problems. Its focus on memory safety, performance, and concurrency makes it an attractive alternative for developing safe and reliable embedded systems. In this blog post, we'll explore how Rust's features make it an ideal language for embedded systems development.
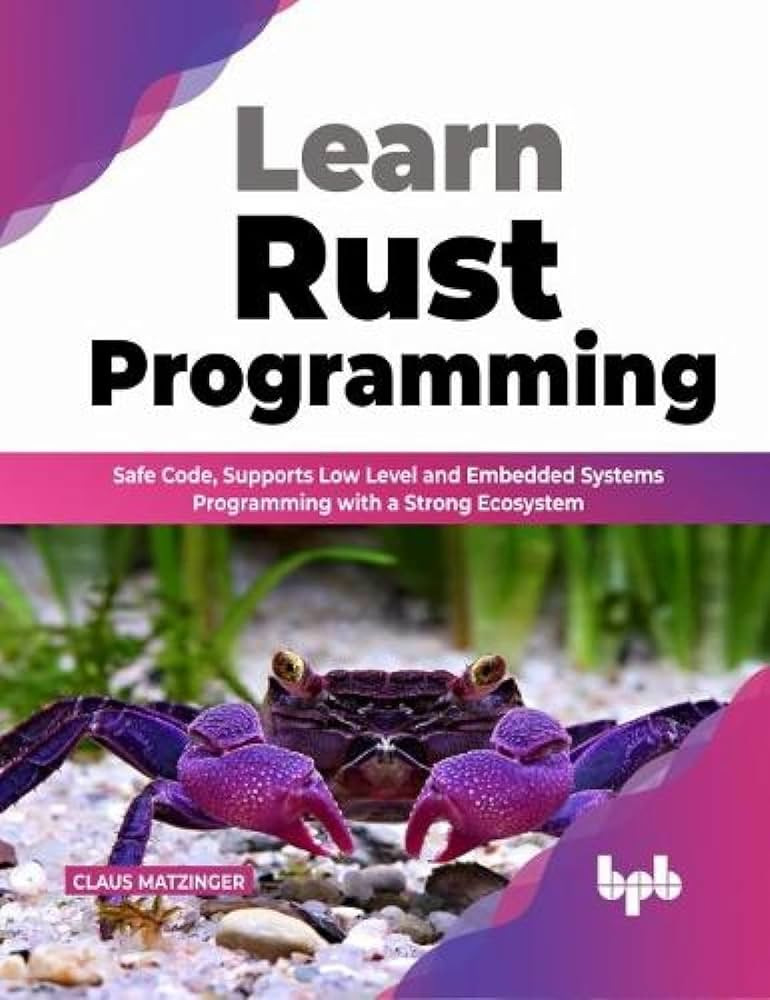
- Memory Safety
- Performance
- Concurrency
- Error Handling
- Embedded Systems Development with Rust
- Practical Example: Blinking an LED with Rust
- Conclusion
- Ready to Start Developing Safe Embedded Systems with Rust?
Memory Safety
Memory safety is a critical concern in embedded systems. C and C++'s lack of memory safety guarantees leads to issues like null pointer dereferences, data races, and buffer overflows. Rust's ownership model and borrow checker ensure memory safety by preventing common errors like:
- Null pointer dereferences
- Data races
- Buffer overflows
- Double-free errors
Rust's ownership model is based on the concept of ownership and borrowing. Each value in Rust has an owner that is responsible for deallocating the memory when it is no longer needed. The borrow checker ensures that there is only one mutable reference or multiple immutable references to a value at any given time.
let mut x = 5; // x is the owner of the value 5
let y = &x; // y is an immutable reference to x
let z = &mut x; // error: cannot borrow x as mutable because it is already borrowed
Performance
Performance is crucial in embedded systems, where resources are limited and latency is critical. Rust's performance is on par with C and C++, thanks to its low-level memory management and lack of runtime overhead.
Rust's abstractions, such as the std::collections
module, are designed to be efficient and provide high-performance data structures. Additionally, Rust's compiler is capable of optimizing code aggressively, resulting in fast execution times.
let mut vec = Vec::new(); // create a vector
vec.push(1); // push an element onto the vector
vec.push(2);
let sum: i32 = vec.into_iter().sum(); // sum the elements of the vector
println!("Sum: {}", sum); // print the sum
Concurrency
Concurrency is essential in modern embedded systems, where multiple tasks need to be executed simultaneously. Rust's concurrency model is based on the concept of ownership and borrowing, which ensures memory safety even in the presence of concurrency.
Rust provides a number of concurrency primitives, such as threads, locks, and atomic variables, which enable developers to write concurrent code safely and efficiently.
use std::thread;
let handle = thread::spawn(|| {
// thread code
println!("Hello from thread!");
});
handle.join().unwrap();
Error Handling
Error handling is critical in embedded systems, where errors can have serious consequences. Rust's error handling system is based on the concept of error types and the Result
enum.
Rust's error handling system ensures that errors are handled explicitly, rather than silently ignoring them. This approach helps developers catch and handle errors early, preventing them from propagating and causing system failures.
use std::fs::File;
let f = File::open("hello.txt");
match f {
Ok(file) => println!("File opened successfully!"),
Err(error) => println!("Error opening file: {}", error),
}
Embedded Systems Development with Rust
Rust is not only a suitable language for embedded systems development but also provides a number of libraries and frameworks that make it easy to develop and deploy embedded systems.
The Rust Embedded Working Group provides a set of libraries and tools for developing embedded systems, including:
- **cortex-m`: A Rust library for developing ARM Cortex-M microcontrollers.
- **panic-halt`: A library for handling panics in embedded systems.
- rust-std: Rust's standard library, which provides a number of useful functions for embedded systems development.
Practical Example: Blinking an LED with Rust
Here's a simple example of how to blink an LED using Rust on an STM32F3DISCOVERY board:
use cortex_m_rt::prelude::*;
fn main() {
let device = stm32f3::Peripherals::take().unwrap();
let gpioe = device.GPIOE.split();
let mut led = gpioe.pe9.into_push_pull_output();
loop {
led.set_high().unwrap();
cortex_m_rt::delay(500);
led.set_low().unwrap();
cortex_m_rt::delay(500);
}
}
Conclusion
In this blog post, we explored how Rust's unique features make it an ideal language for developing safe and reliable embedded systems. Rust's focus on memory safety, performance, and concurrency makes it an attractive alternative to C and C++ for embedded systems development.
By leveraging Rust's features and libraries, developers can create robust, efficient, and safe embedded systems that meet the demanding requirements of modern applications.
Ready to Start Developing Safe Embedded Systems with Rust?
Start exploring Rust's features and libraries today and discover how it can help you develop safe and reliable embedded systems.
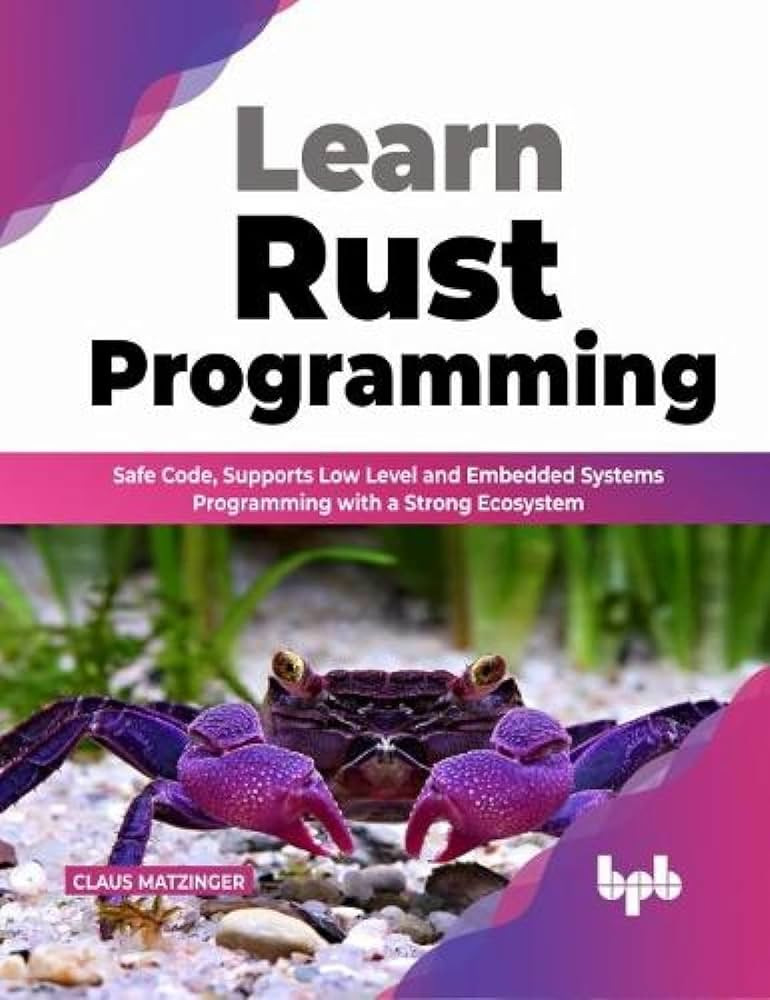