- Published on
Building a Local Blockchain From Theory to Implementation
- Authors
- Name
- Adil ABBADI
Introduction
Blockchain technology has revolutionized the way we think about data storage and transmission. Initially introduced as the underlying technology behind Bitcoin, blockchain has evolved to become a decentralized, distributed ledger technology with far-reaching applications beyond digital currencies. In this blog post, we'll embark on a journey to build a local blockchain from scratch using Python, covering the theory and implementation of this groundbreaking technology.
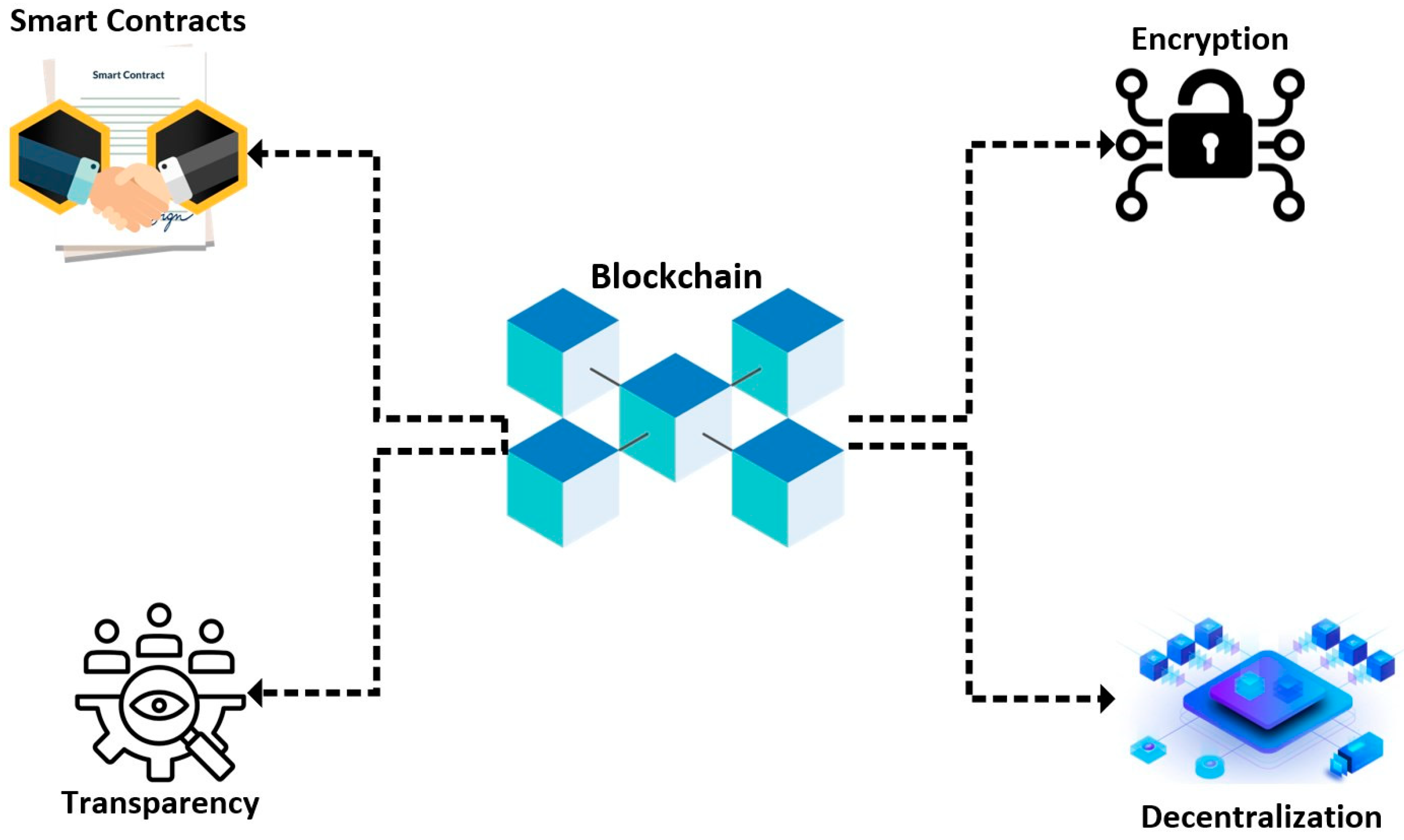
- Understanding Blockchain Fundamentals
- Building the Local Blockchain
- Simulation: Multiple Nodes and Consensus
- Conclusion
Understanding Blockchain Fundamentals
Before diving into the implementation, let's revisit the fundamental concepts that make blockchain technology tick:
- Decentralization: A blockchain is a decentralized system, meaning that there is no central authority controlling the network. Instead, a network of nodes verifies and validates transactions.
- Immutable Ledger: The blockchain is an immutable ledger, where once a transaction is written, it cannot be altered or deleted.
- Consensus Mechanism: Nodes on the network use a consensus mechanism to agree on the state of the blockchain, ensuring that all nodes have the same version of the ledger.
- Mining: In Proof-of-Work (PoW) blockchain networks, miners compete to solve complex mathematical puzzles to validate transactions and add new blocks to the chain.
Building the Local Blockchain
We'll use Python to build a simplified local blockchain, focusing on the core components:
1. Block Class
A block represents a single unit of data in the blockchain. Create a Block
class to define the structure of a block:
import hashlib
import time
class Block:
def __init__(self, index, previous_hash, timestamp, data):
self.index = index
self.previous_hash = previous_hash
self.timestamp = timestamp
self.data = data
self.hash = self.calculate_hash()
def calculate_hash(self):
data_string = str(self.index) + self.previous_hash + str(self.timestamp) + str(self.data)
return hashlib.sha256(data_string.encode()).hexdigest()
2. Blockchain Class
The Blockchain
class will manage the chain of blocks and provide methods for adding new blocks:
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block(0, "0", int(time.time()), "Genesis Block")
def add_block(self, new_block):
new_block.previous_hash = self.chain[-1].hash
new_block.hash = new_block.calculate_hash()
self.chain.append(new_block)
3. Node Class
A node represents a single entity on the network. Create a Node
class to manage the blockchain and communicate with other nodes:
class Node:
def __init__(self, blockchain):
self.blockchain = blockchain
def add_block(self, block):
self.blockchain.add_block(block)
def synchronize_chain(self, other_node):
if len(self.blockchain.chain) < len(other_node.blockchain.chain):
self.blockchain.chain = other_node.blockchain.chain
4. Running the Local Blockchain
Create a local node and add some blocks to the chain:
node = Node(Blockchain())
# Add some blocks
node.add_block(Block(1, node.blockchain.chain[-1].hash, int(time.time()), "Block 1"))
node.add_block(Block(2, node.blockchain.chain[-1].hash, int(time.time()), "Block 2"))
node.add_block(Block(3, node.blockchain.chain[-1].hash, int(time.time()), "Block 3"))
Simulation: Multiple Nodes and Consensus
To simulate a decentralized network, we'll create multiple nodes and implement a basic consensus mechanism using a voting system:
# Create multiple nodes
node1 = Node(Blockchain())
node2 = Node(Blockchain())
node3 = Node(Blockchain())
# Add blocks to each node
node1.add_block(Block(1, node1.blockchain.chain[-1].hash, int(time.time()), "Block 1"))
node2.add_block(Block(1, node2.blockchain.chain[-1].hash, int(time.time()), "Block 1"))
node3.add_block(Block(1, node3.blockchain.chain[-1].hash, int(time.time()), "Block 1"))
# Simulate consensus
def consensus(nodes):
max_chain_length = 0
longest_chain = None
for node in nodes:
if len(node.blockchain.chain) > max_chain_length:
max_chain_length = len(node.blockchain.chain)
longest_chain = node.blockchain.chain
return longest_chain
# Synchronize nodes
nodes = [node1, node2, node3]
longest_chain = consensus(nodes)
for node in nodes:
node.blockchain.chain = longest_chain
Conclusion
In this blog post, we embarked on a journey to build a local blockchain from scratch using Python, covering the fundamental concepts and implementation details of a decentralized, distributed ledger technology. While this is a simplified example, it demonstrates the core principles of blockchain technology and provides a solid foundation for further exploration and development.
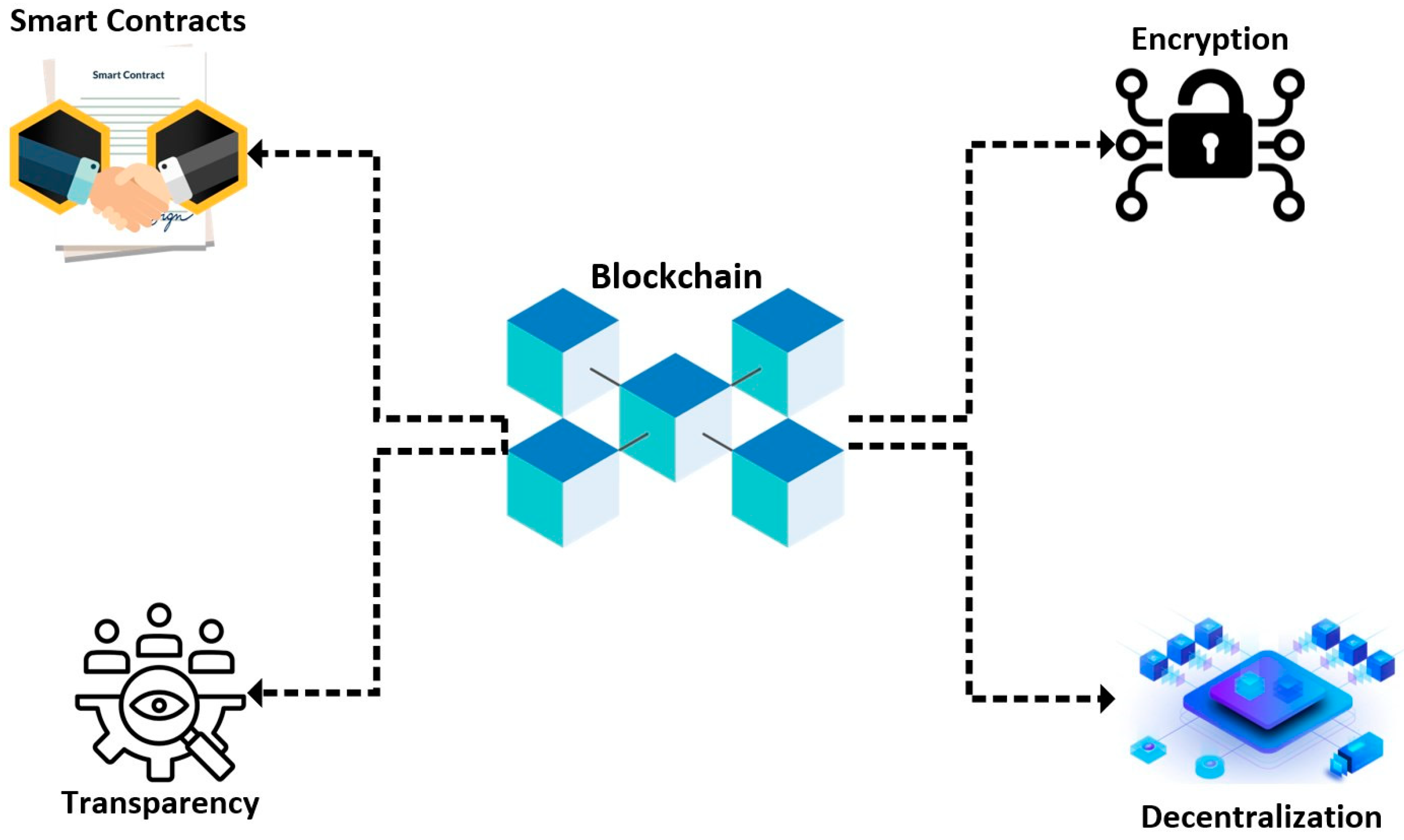
Ready to Take Your Blockchain Skills to the Next Level?
Start building your own blockchain applications today and explore the vast possibilities of decentralized technology!
Remember to stay tuned for more in-depth tutorials and projects on blockchain development!