- Published on
Building a Local AI Chatbot with Open Source LLMs
- Authors
- Name
- Adil ABBADI
Introduction
The rise of conversational AI has led to a surge in the development of chatbots that can understand and respond to human language. While many chatbot platforms rely on cloud-based services, building a local AI chatbot can provide greater control, security, and customization. In this blog post, we will explore how to build a local AI chatbot using open source large language models (LLMs).
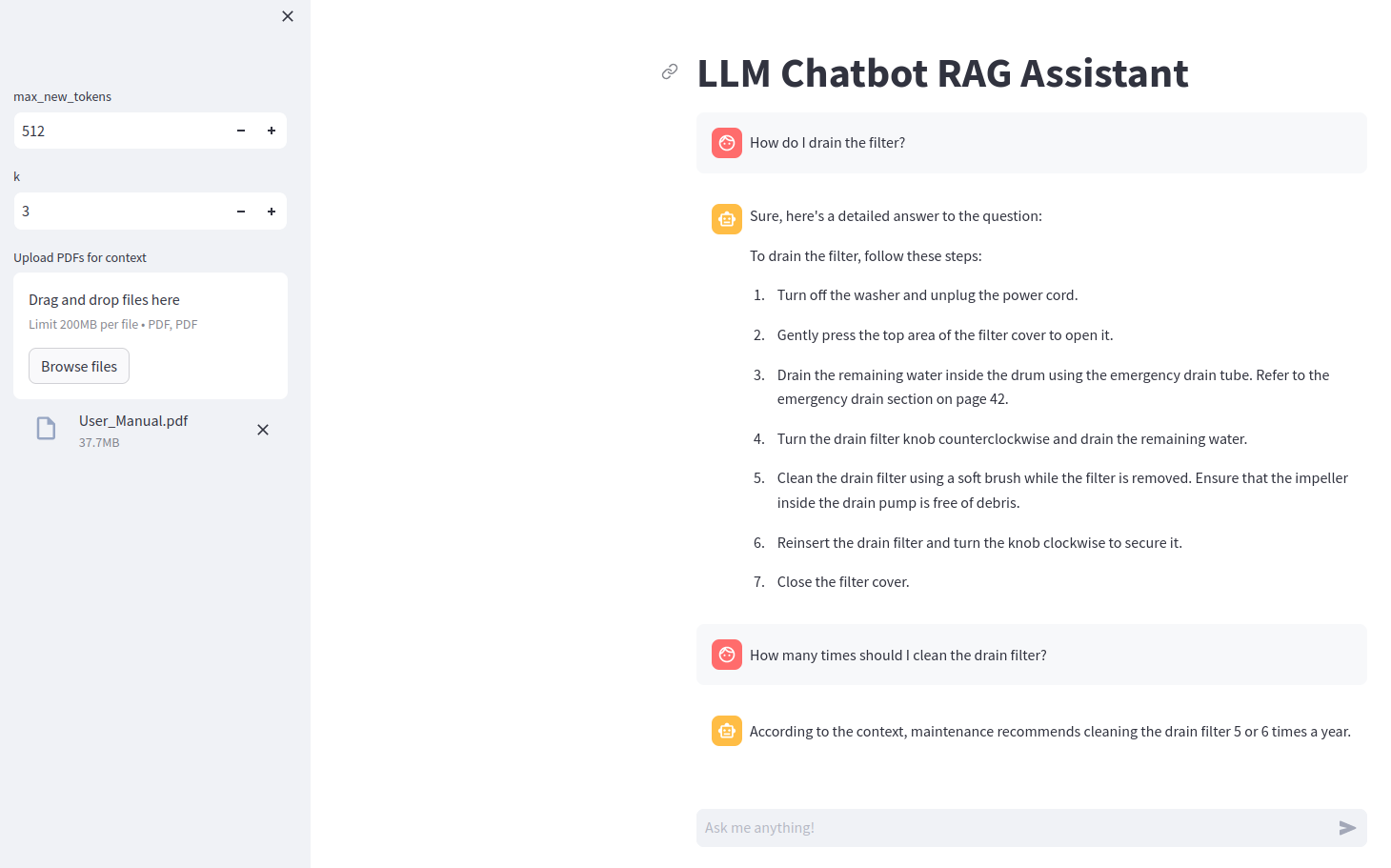
- Why Open Source LLMs?
- Choosing the Right Open Source LLM
- Building the Chatbot
- Deploying the Chatbot
- Conclusion
- Ready to Build Your Own Chatbot?
Why Open Source LLMs?
Open source LLMs offer a cost-effective and customizable alternative to proprietary models. They can be fine-tuned for specific tasks and domains, allowing for greater flexibility and control. Moreover, open source LLMs can be deployed locally, reducing reliance on cloud services and ensuring data privacy.
Choosing the Right Open Source LLM
Several open source LLMs are available, each with its strengths and weaknesses. For this example, we will use the Hugging Face Transformers library, which provides a wide range of pre-trained models.
Hugging Face Transformers
Hugging Face is a popular open source library that provides a wide range of pre-trained language models, including BERT, RoBERTa, and DistilBERT. The library is built on top of PyTorch and provides an easy-to-use interface for fine-tuning and deploying models.
pip install transformers
Model Selection
For this example, we will use the DistilBERT model, which is a smaller and more efficient version of BERT.
from transformers import DistilBertTokenizer, DistilBertForSequenceClassification
Building the Chatbot
Our chatbot will consist of the following components:
- Natural Language Processing (NLP) Module: responsible for processing user input and generating responses.
- Knowledge Graph: stores domain-specific knowledge and information.
- Integration Layer: integrates the NLP module and knowledge graph to generate responses.
NLP Module
The NLP module will use the DistilBERT model to process user input and generate responses.
import torch
from transformers import DistilBertTokenizer, DistilBertForSequenceClassification
# Load pre-trained DistilBERT model and tokenizer
tokenizer = DistilBertTokenizer.from_pretrained('distilbert-base-uncased')
model = DistilBertForSequenceClassification.from_pretrained('distilbert-base-uncased')
def process_input(input_text):
# Tokenize input text
inputs = tokenizer.encode_plus(input_text,
add_special_tokens=True,
max_length=512,
return_attention_mask=True,
return_tensors='pt')
# Forward pass
output = model(inputs['input_ids'], attention_mask=inputs['attention_mask'])
# Get generated response
response = output.logits.argmax(-1).item()
return response
Knowledge Graph
The knowledge graph will store domain-specific knowledge and information.
import networkx as nx
# Create a knowledge graph using NetworkX
G = nx.Graph()
# Add nodes and edges to the graph
G.add_node('Node 1', description='This is node 1')
G.add_node('Node 2', description='This is node 2')
G.add_edge('Node 1', 'Node 2', relation='Related to')
def get_response_from_kg(input_text):
# Query the knowledge graph using the input text
response = nx.get_node_attributes(G, 'description')[input_text]
return response
Integration Layer
The integration layer will integrate the NLP module and knowledge graph to generate responses.
def generate_response(input_text):
# Process input text using the NLP module
nlp_response = process_input(input_text)
# Query the knowledge graph using the NLP response
kg_response = get_response_from_kg(nlp_response)
# Combine responses
response = f'{nlp_response} {kg_response}'
return response
Deploying the Chatbot
Once the chatbot is built, it can be deployed using a variety of platforms and frameworks, such as Flask, Django, or React. For this example, we will use Flask to create a simple web interface for the chatbot.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/chat', methods=['POST'])
def chat():
input_text = request.json['input_text']
response = generate_response(input_text)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Conclusion
In this blog post, we explored how to build a local AI chatbot using open source large language models. By leveraging the Hugging Face Transformers library and fine-tuning the DistilBERT model, we can create a highly accurate and efficient chatbot. The chatbot can be deployed using a variety of platforms and frameworks, providing a flexible and customizable solution for conversational AI applications.
Ready to Build Your Own Chatbot?
Start building your own local AI chatbot today and explore the possibilities of open source LLMs.