- Published on
Rust vs C++ Memory Safety in Systems Programming
- Authors
- Name
- Adil ABBADI
Introduction
Systems programming is a critical aspect of computer science, involving the development of operating systems, device drivers, and other low-level system software. Two popular languages for systems programming are Rust and C++. While both languages share some similarities, they differ significantly in their approach to memory safety. In this post, we'll explore the differences between Rust and C++ and why Rust is gaining popularity as a safer alternative for systems programming.
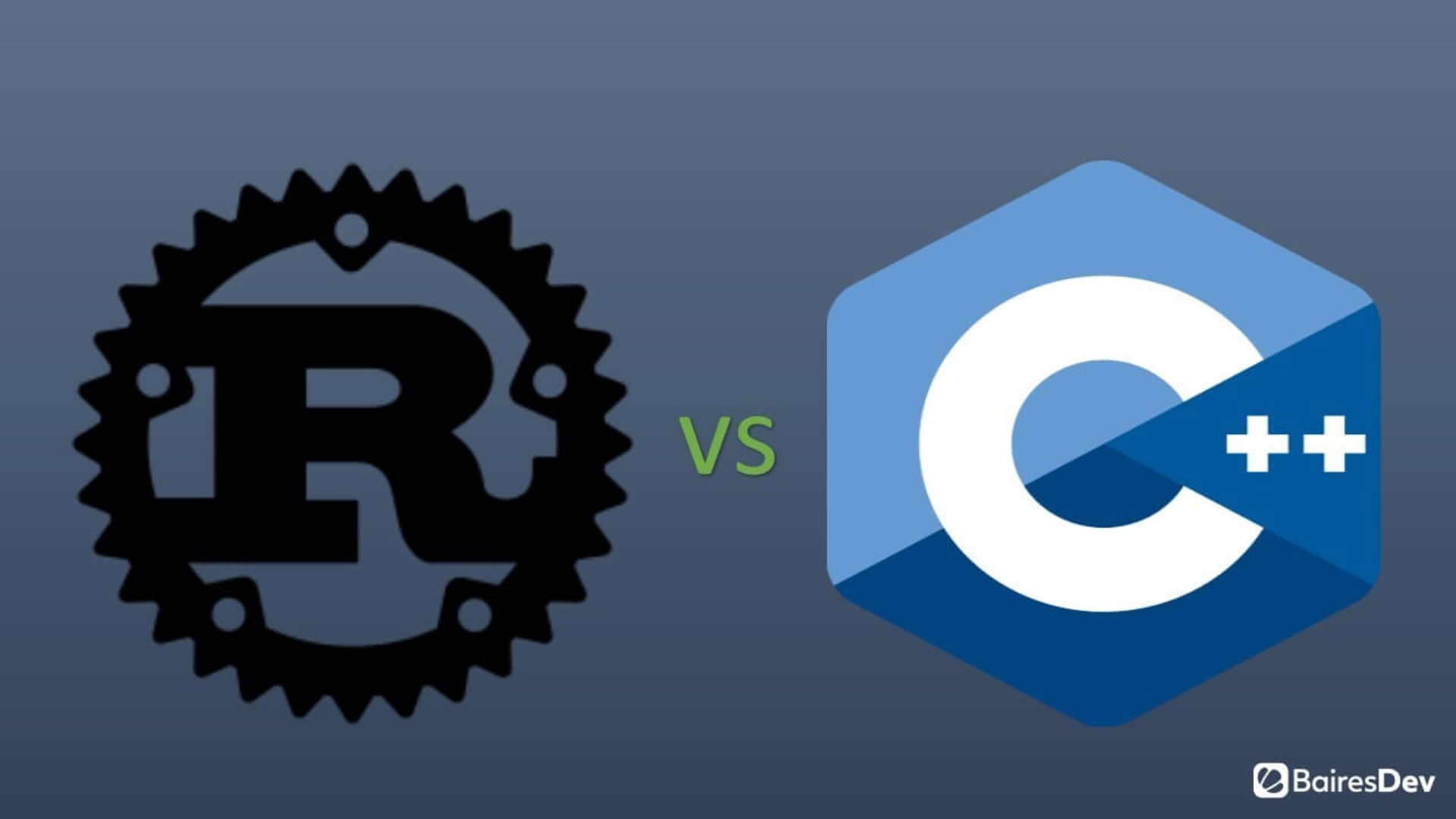
- Memory Safety: The Achilles' Heel of C++
- Rust: The Memory-Safe Alternative
- Performance Comparison
- Conclusion
- Ready to Switch to Rust?
Memory Safety: The Achilles' Heel of C++
Memory safety is a critical aspect of systems programming, as it directly affects the reliability and security of the system. C++, a powerful and performance-oriented language, is notorious for its lack of memory safety features. C++'s memory management model relies on manual memory allocation and deallocation using pointers, which can lead to:
- Buffer Overflows: Writing beyond the boundaries of an array can corrupt adjacent memory regions.
- Dangling Pointers: Pointers that reference memory locations that have already been freed or reused.
- Use-After-Free: Accessing memory regions after they have been deallocated.
- Data Corruption: Modifying unintended parts of the memory, leading to data corruption.
These vulnerabilities can be exploited by attackers, leading to security breaches and system crashes.
// Example of manual memory allocation in C++
int* ptr = new int;
*ptr = 10;
delete ptr;
Rust: The Memory-Safe Alternative
Rust, a relatively new language, takes a different approach to memory safety. It introduces a concept called Ownership, which ensures that each value has a single owner responsible for managing its memory. This approach eliminates the need for manual memory management and prevents common memory safety issues.
Rust's ownership model is based on three principles:
- Ownership: Each value has a single owner that manages its memory.
- Borrowing: Values can be borrowed, but only one mutable or multiple immutable borrows are allowed at a time.
- ** Lifetimes**: The lifetime of a reference is ensured by the compiler, preventing dangling pointers.
Rust's compile-time checks and runtime checks ensure that memory safety issues are caught early, preventing common vulnerabilities.
// Example of memory safety in Rust
let mut x = 10;
let y = &mut x; // mutable borrow
*y = 20;
println!("{}", x); // prints 20
Performance Comparison
A common misconception is that Rust's memory safety features come at the cost of performance. However, Rust's abstractions and compiler optimizations ensure that the performance difference between Rust and C++ is minimal.
In some cases, Rust's performance even surpasses C++ due to its ability to optimize memory management and prevent unnecessary copies.
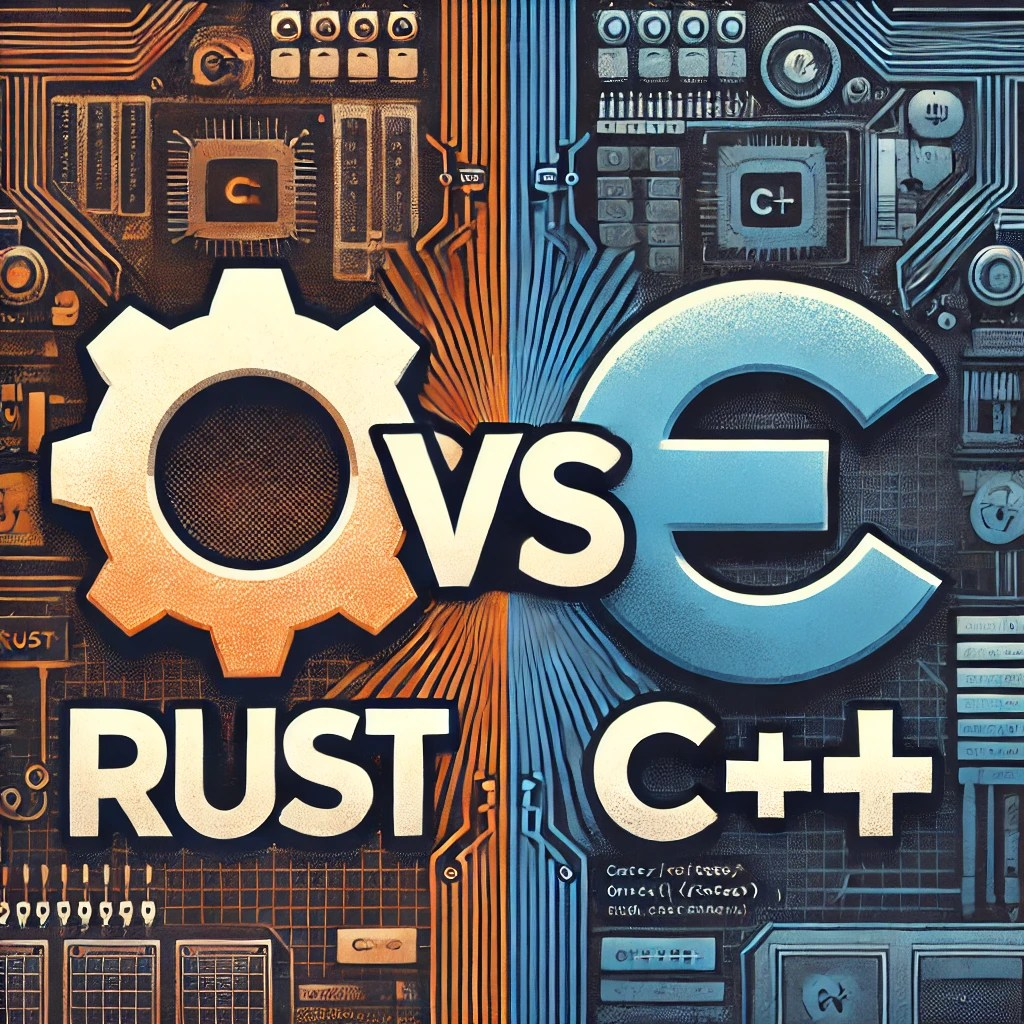
Conclusion
In conclusion, Rust offers a safer and more reliable alternative to C++ for systems programming. Its ownership model and borrow checker ensure memory safety, preventing common vulnerabilities and security breaches. While C++ remains a powerful language, Rust's modern design and safety features make it an attractive choice for systems programming.
Ready to Switch to Rust?
Start exploring Rust today and discover the benefits of memory-safe systems programming.
Further Reading